
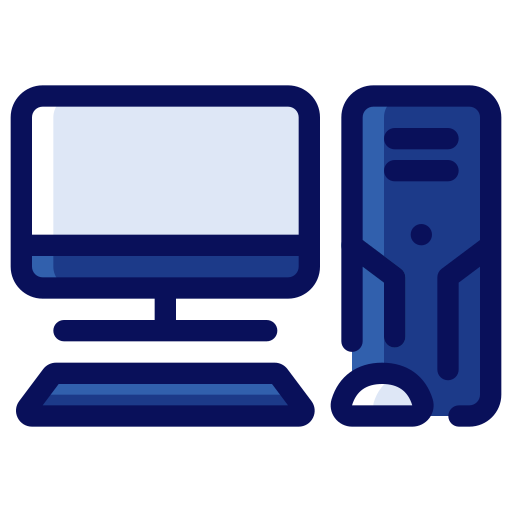
Kotaku is shit and lacks a vision other than writing a bunch of polarizing counter culture bullshit and shouldnt be trusted to factually report on anything.
Kotaku is shit and lacks a vision other than writing a bunch of polarizing counter culture bullshit and shouldnt be trusted to factually report on anything.
Removed by mod
So you just don’t buy anything? Get over yourself and your unhealthy obsessions.
Ethernet is the common PHY even across fiber afaik.
fossfreaks strike again
This behavior is simple, it just wasn’t explained to you at the correct level.
All blocks (code between {
and }
) evaluate to some value, they’re all treated as expressions. A function “without a return type” will always return ()
because that’s what a block that doesn’t have any “output” “returns”.
This understanding is fundamental to Rust and, for example, is involved in understanding when we have to and don’t have to use return
in a function. Quite usefully, it also allows us to organize our code by creating a new scope, i.e.
let foo: u32 = {
// Hate dropping mutex guards explicitly? This is one way you can avoid that (although it's arguably a code smell that I discourage and should not have even mentioned)
// This block evaluates to 100
100
};
let foo: () = {
println!("this block evaluates to the unit type");
}
The way Rust interprets the issue with your function is:
()
because it doesn’t have an explicit return valuei32
which is not ()
The reason the error is perhaps more unclear than it could be is because they assume you have read the Rust book or understand how blocks are expressions themselves.
It would be valuable to look at https://doc.rust-lang.org/book/ch03-03-how-functions-work.html for more details and to gain a more thorough understanding of the topic at hand. I left out the precise details on “which expression from the block is the one that it evaluates to” other than the obvious case of “the very last expression”.
I’ll do you one better!
let foo: [u8; 10] = Default::default();
(pretty sure the above only works reliably after they finally were able to derive traits on n-sized arrays but that’s been in for a while now)
And as you suggested:
let foo: [u8; 10] = [Default::default(); 10];
f32
is a type, you need to actually provide a value to the shorthand array initialization syntax, the array is filled with that value. There is no such thing as “uninitialized” or implicit zeroing here.
let foo: [f32; 5] = [0.0; 5];
You seem to have indicated dismay over the default f64 type.
To type a integer or float literal, suffix it with its built-in type. For example:
0.0f32
1024usize
Does this look ugly? Yes. Good news! It’s hardly relevant in an actual project because Rust will easily default to whichever float type you are using throughout the project as your floating point numbers with resolved types will resolve the types of the implicitly typed floating point numbers.
You can also use the same syntax with the vec![]
macro :)
let bar = vec![0.0f32; 5];
The true connector crime here is that single USB-C, any new PC should have more than that.
Yeah… even the expensive motherboards Ive bought have like two max, it sucks
Soo… more tasks to not be able to do? Seems pretty genius.
See ya in two years when AI is dead and mozilla is pivoting to “focus on the fundamentals” and only works on the browser
Until Zigbee2MQTT breaks again ;P
What the fuck are you talking about? This garbled comment deserves the cesspool.
Wish granted, you’re now mute because he sure doesn’t sound like much anymore ;)
Good thing the world is that simple, you’re completely correct. Nobody who could theoretically prevent something they don’t like is not entitled to their dislike, duh!
I wont use all caps but they are indeed all over the netherlands. This discussion is hella lacking context. Yes those racks are very common and normal, and no amount of what you think changes that. This is a dutch ad which is based on what NL actually looks like.
Acting like the 80s were a new time for bikes… by then the netherlands was not occupied and the dutch were indeed allowed to own bikes again :)
Yeah it really sucked, slightly muffled by the mask and no lips to read.
The ways they try to justify ad blockers as their god given right is equally frustrating. It’s okay to use an ad blocker (and you should!). Please stop acting personally insulted when sites then attempt to make your ad blocker useless, it’s just how things go.
Advertising is horrible
Yeah I mean closed captions, oops
I’d imagine the fact that is not legal and is negligent would stop them.